Using GraphQL Typegen with Gatsby
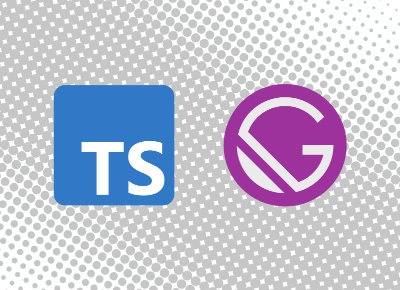
Table of Contents
§ Using GraphQL Typegen
Upon creating a JavaScript codebase in TypeScript I want to stay true to adding all type definitions, but I'm the first to tell you there are some times it's just so tedious I end up using variable: any
. This is particularly true if the object is a query, especially if there's something that automates it, right?
It turns out Gatsby has a good feature called GraphQL Typegen just for this circumstance. If you've arrived here, chances are you've already gone through the documentation on Gatsby's website. I followed the instructions, but had trouble with one part of the whole procedure that made all the difference.
The first thing of course, is setting graphqlTypegen
to true.
// gatsby-config.ts
module.exports = {
graphqlTypegen: true,
}
The instructions should also let you know to add the autogenerated file (gatsby-types.d.ts
) name to your tsconfig.json
file.
// tsconfig.json
{
"compilerOptions": {
"target": "esnext",
"lib": ["dom", "esnext"],
"jsx": "react-jsx",
"module": "esnext",
"baseUrl": "src",
"moduleResolution": "node",
"esModuleInterop": true,
"resolveJsonModule": true,
"inlineSources": true,
"forceConsistentCasingInFileNames": true,
"strict": false,
"sourceMap": true,
"sourceRoot": "/",
"skipLibCheck": true,
"paths": {
"@/*": ["./src/*"],
"@/static/*": ["./static/*"]
},
"types": ["node", "@testing-library/jest-dom"]
},
"exclude": ["node_modules"],
"include": [
"./src",
"./src/**/*",
"./gatsby-node.ts",
"./gatsby-config.ts",
"./plugins/**/*",
".d.ts",
"gatsby-types.d.ts"
]
}
The one caveat? You have to name all your queries, like this:
query Alpine {
publishedPosts: allMdx(
sort: { frontmatter: { date: ASC } }
filter: { frontmatter: { status: { in: ["published"] } } }
) {
nodes {
id
frontmatter {
tags
}
fields {
slug
}
internal {
contentFilePath
}
}
}
If you miss naming one of your queries, or adopt the same name for two or more, you will get an error.
This file only generates during gatsby develop
. Once this file is generated you will be able to use autocomplete to type the results of your queries.
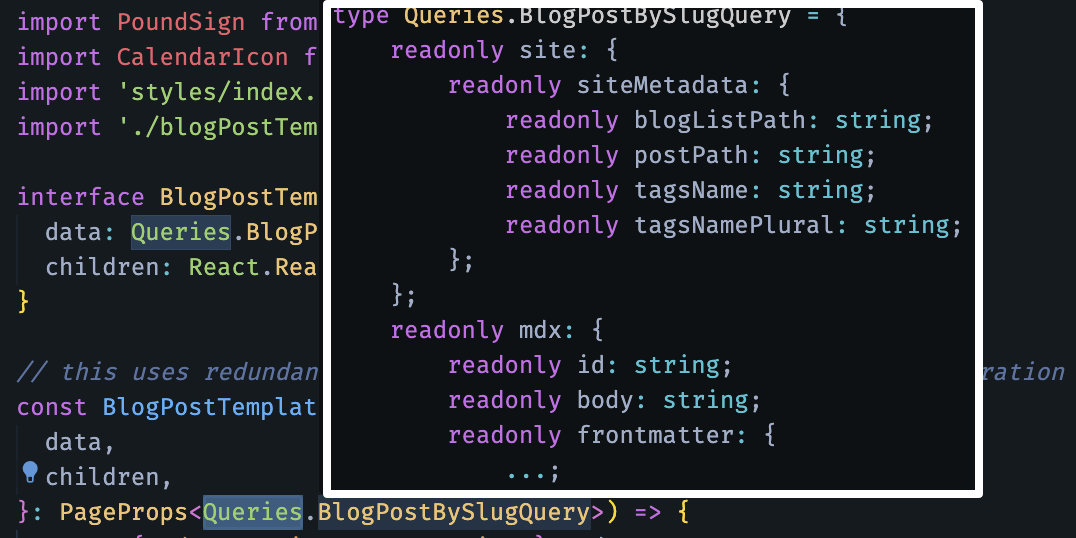