Absolute Paths with React and Webpack
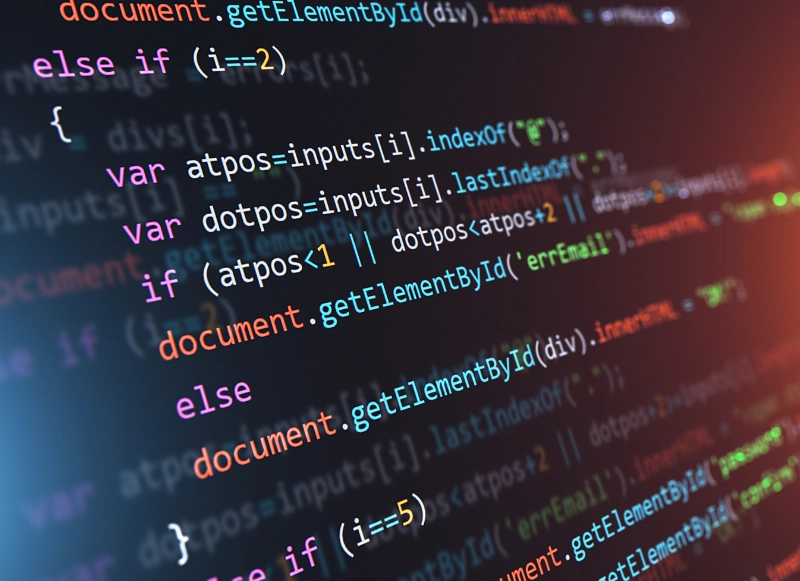
Table of Contents
§ Relative Paths are Confusing
How many directories deep is the component you are working with? If your code is littered with import
paths like this, you are definitely looking for relief.
import { useSiteMetadata } from '../hooks/use-site-metadata';
import CalendarIcon from '../../assets/calendar.svg';
import DoubleChevronArrow from '../../assets/double-chevron.svg';
import '../../../../ArtElement.scss';
This is definitely not a one size fits all solution, but it's worth documenting since I often start with a fresh repository or I am using a new version of X component, and I need to know how to get my absolute paths back.
§ Typescript Settings
The first item is to add a baseUrl
setting for your root directory, which in this case is src
. You must also list src
as an include
.
// tsconfig.json
{
"compilerOptions": {
"jsx": "react-jsx",
"target": "es6",
"esModuleInterop": true,
"allowSyntheticDefaultImports": true,
"forceConsistentCasingInFileNames": true,
"strict": false,
"skipLibCheck": true,
"outDir": "dist",
"moduleResolution": "node",
"baseUrl": "src" },
"include": ["src", ".d.ts"]}
§ Webpack Settings
I'm always tricked into thinking this is all I need, but when I start the Webpack development server, I get a nasty error message that it cannot find my component. So now we must go into webpack.config.ts
and make sure we account for it in the resolve: {modules:
section.
// webpack.config.ts
resolve: {
modules: ['src', 'node_modules'], extensions: ['*', '.tsx', '.ts', '.js', '.jsx', '.css', '.scss'],
},
That should be it. From now on you are free to add components with wonderful absolute paths like so:
import React from 'react';
import MapTest from 'components/MapTest';